Routes
Requests sent to Strapi on any URL are handled by routes. By default, Strapi generates routes for all the content-types (see REST API documentation). Routes can be added and configured:
- with policies, which are a way to block access to a route,
- and with middlewares, which are a way to control and change the request flow and the request itself.
Once a route exists, reaching it executes some code handled by a controller (see controllers documentation). To view all existing routes and their hierarchal order, you can run yarn strapi routes:list
(see CLI reference).
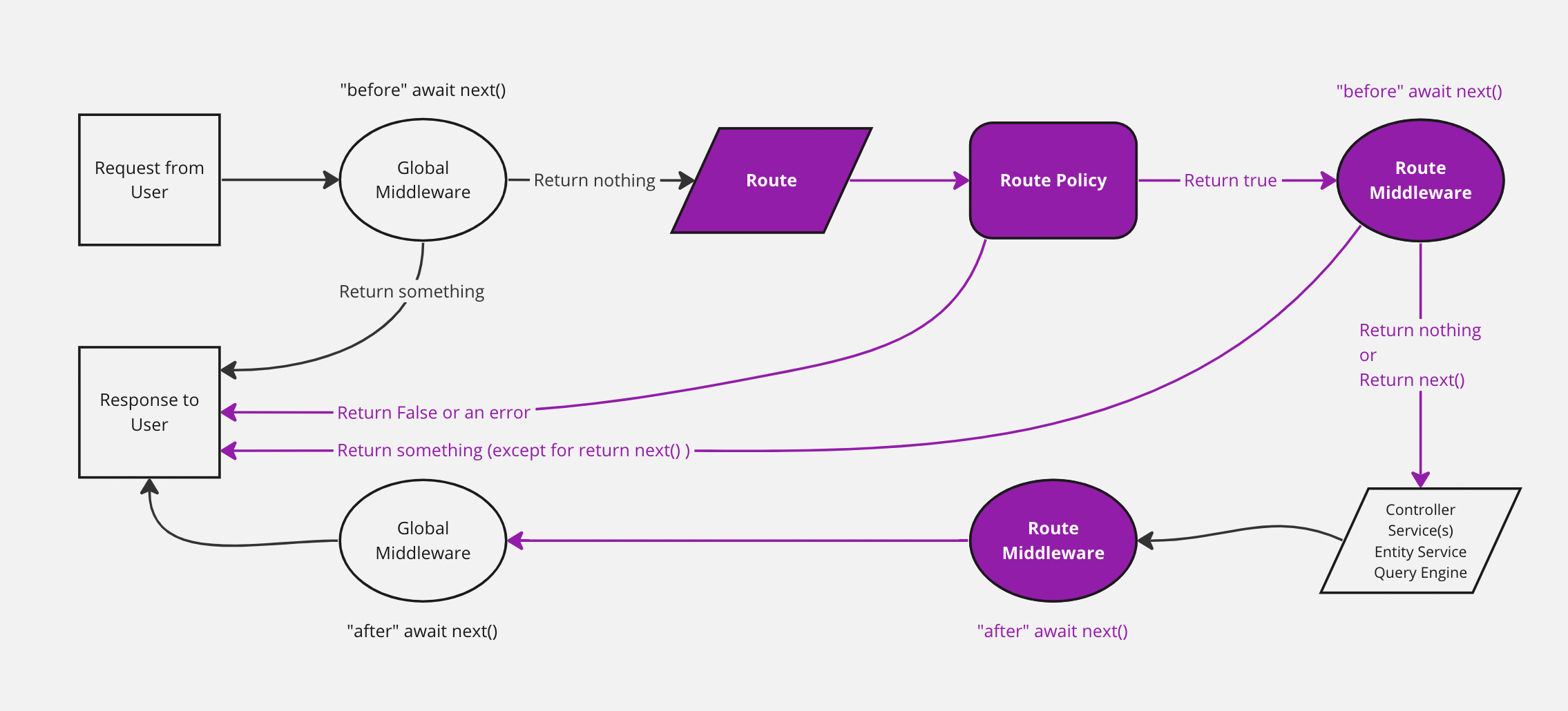
Implementation
Implementing a new route consists in defining it in a router file within the ./src/api/[apiName]/routes
folder (see project structure).
There are 2 different router file structures, depending on the use case:
- configuring core routers
- or creating custom routers.
Configuring core routers
Core routers (i.e. find
, findOne
, create
, update
, and delete
) correspond to default routes automatically created by Strapi when a new content-type is created.
Strapi provides a createCoreRouter
factory function that automatically generates the core routers and allows:
- passing in configuration options to each router
- and disabling some core routers to create custom ones.
A core router file is a JavaScript file exporting the result of a call to createCoreRouter
with the following parameters:
Parameter | Description | Type |
---|---|---|
prefix | Allows passing in a custom prefix to add to all routers for this model (e.g. /test ) | String |
only | Core routes that will only be loaded Anything not in this array is ignored. | Array |
except | Core routes that should not be loaded This is functionally the opposite of the only parameter. | Array |
config | Configuration to handle policies, middlewares and public availability for the route | Object |
- JavaScript
- TypeScript
const { createCoreRouter } = require('@strapi/strapi').factories;
module.exports = createCoreRouter('api::restaurant.restaurant', {
prefix: '',
only: ['find', 'findOne'],
except: [],
config: {
find: {
auth: false,
policies: [],
middlewares: [],
},
findOne: {},
create: {},
update: {},
delete: {},
},
});
import { factories } from '@strapi/strapi';
export default factories.createCoreRouter('api::restaurant.restaurant', {
prefix: '',
only: ['find', 'findOne'],
except: [],
config: {
find: {
auth: false,
policies: [],
middlewares: [],
},
findOne: {},
create: {},
update: {},
delete: {},
},
});
Generic implementation example:
- JavaScript
- TypeScript
const { createCoreRouter } = require('@strapi/strapi').factories;
module.exports = createCoreRouter('api::restaurant.restaurant', {
only: ['find'],
config: {
find: {
auth: false,
policies: [],
middlewares: [],
}
}
});
import { factories } from '@strapi/strapi';
export default factories.createCoreRouter('api::restaurant.restaurant', {
only: ['find'],
config: {
find: {
auth: false,
policies: [],
middlewares: [],
}
}
});
This only allows a GET
request on the /restaurants
path from the core find
controller without authentication.
Creating custom routers
Creating custom routers consists in creating a file that exports an array of objects, each object being a route with the following parameters:
Parameter | Description | Type |
---|---|---|
method | Method associated to the route (i.e. GET , POST , PUT , DELETE or PATCH ) | String |
path | Path to reach, starting with a forward-leading slash (e.g. /articles ) | String |
handler | Function to execute when the route is reached. Should follow this syntax: <controllerName>.<actionName> | String |
config Optional | Configuration to handle policies, middlewares and public availability for the route | Object |
Dynamic routes can be created using parameters and regular expressions. These parameters will be exposed in the ctx.params
object. For more details, please refer to the PathToRegex documentation.
Routes files are loaded in alphabetical order. To load custom routes before core routes, make sure to name custom routes appropriately (e.g. 01-custom-routes.js
and 02-core-routes.js
).
Example of a custom router using URL parameters and regular expressions for routes
In the following example, the custom routes file name is prefixed with 01-
to make sure the route is reached before the core routes.
- JavaScript
- TypeScript
module.exports = {
routes: [
{ // Path defined with an URL parameter
method: 'POST',
path: '/restaurants/:id/review',
handler: 'restaurant.review',
},
{ // Path defined with a regular expression
method: 'GET',
path: '/restaurants/:category([a-z]+)', // Only match when the URL parameter is composed of lowercase letters
handler: 'restaurant.findByCategory',
}
]
}
export default {
routes: [
{ // Path defined with a URL parameter
method: 'GET',
path: '/restaurants/:category/:id',
handler: 'Restaurant.findOneByCategory',
},
{ // Path defined with a regular expression
method: 'GET',
path: '/restaurants/:region(\\d{2}|\\d{3})/:id', // Only match when the first parameter contains 2 or 3 digits.
handler: 'Restaurant.findOneByRegion',
}
]
}
Configuration
Both core routers and custom routers have the same configuration options. The routes configuration is defined in a config
object that can be used to handle policies and middlewares or to make the route public.
Policies
Policies can be added to a route configuration:
- by pointing to a policy registered in
./src/policies
, with or without passing a custom configuration - or by declaring the policy implementation directly, as a function that takes
policyContext
to extend Koa's context (ctx
) and thestrapi
instance as arguments (see policies documentation)
- Core router policy
- Custom router policy
- JavaScript
- TypeScript
const { createCoreRouter } = require('@strapi/strapi').factories;
module.exports = createCoreRouter('api::restaurant.restaurant', {
config: {
find: {
policies: [
// point to a registered policy
'policy-name',
// point to a registered policy with some custom configuration
{ name: 'policy-name', config: {} },
// pass a policy implementation directly
(policyContext, config, { strapi }) => {
return true;
},
]
}
}
});
import { factories } from '@strapi/strapi';
export default factories.createCoreRouter('api::restaurant.restaurant', {
config: {
find: {
policies: [
// point to a registered policy
'policy-name',
// point to a registered policy with some custom configuration
{ name: 'policy-name', config: {} },
// pass a policy implementation directly
(policyContext, config, { strapi }) => {
return true;
},
]
}
}
});
- JavaScript
- TypeScript
module.exports = {
routes: [
{
method: 'GET',
path: '/articles/customRoute',
handler: 'api::api-name.controllerName.functionName', // or 'plugin::plugin-name.controllerName.functionName' for a plugin-specific controller
config: {
policies: [
// point to a registered policy
'policy-name',
// point to a registered policy with some custom configuration
{ name: 'policy-name', config: {} },
// pass a policy implementation directly
(policyContext, config, { strapi }) => {
return true;
},
]
},
},
],
};
export default {
routes: [
{
method: 'GET',
path: '/articles/customRoute',
handler: 'api::api-name.controllerName.functionName', // or 'plugin::plugin-name.controllerName.functionName' for a plugin-specific controller
config: {
policies: [
// point to a registered policy
'policy-name',
// point to a registered policy with some custom configuration
{ name: 'policy-name', config: {} },
// pass a policy implementation directly
(policyContext, config, { strapi }) => {
return true;
},
]
},
},
],
};
Middlewares
Middlewares can be added to a route configuration:
- by pointing to a middleware registered in
./src/middlewares
, with or without passing a custom configuration - or by declaring the middleware implementation directly, as a function that takes Koa's context (
ctx
) and thestrapi
instance as arguments:
- Core router middleware
- Custom router middleware
- JavaScript
- TypeScript
const { createCoreRouter } = require('@strapi/strapi').factories;
module.exports = createCoreRouter('api::restaurant.restaurant', {
config: {
find: {
middlewares: [
// point to a registered middleware
'middleware-name',
// point to a registered middleware with some custom configuration
{ name: 'middleware-name', config: {} },
// pass a middleware implementation directly
(ctx, next) => {
return next();
},
]
}
}
});
import { factories } from '@strapi/strapi';
export default factories.createCoreRouter('api::restaurant.restaurant', {
config: {
find: {
middlewares: [
// point to a registered middleware
'middleware-name',
// point to a registered middleware with some custom configuration
{ name: 'middleware-name', config: {} },
// pass a middleware implementation directly
(ctx, next) => {
return next();
},
]
}
}
});
- JavaScript
- TypeScript
module.exports = {
routes: [
{
method: 'GET',
path: '/articles/customRoute',
handler: 'api::api-name.controllerName.functionName', // or 'plugin::plugin-name.controllerName.functionName' for a plugin-specific controller
config: {
middlewares: [
// point to a registered middleware
'middleware-name',
// point to a registered middleware with some custom configuration
{ name: 'middleware-name', config: {} },
// pass a middleware implementation directly
(ctx, next) => {
return next();
},
],
},
},
],
};
export default {
routes: [
{
method: 'GET',
path: '/articles/customRoute',
handler: 'api::api-name.controllerName.functionName', // or 'plugin::plugin-name.controllerName.functionName' for a plugin-specific controller
config: {
middlewares: [
// point to a registered middleware
'middleware-name',
// point to a registered middleware with some custom configuration
{ name: 'middleware-name', config: {} },
// pass a middleware implementation directly
(ctx, next) => {
return next();
},
],
},
},
],
};
Public routes
By default, routes are protected by Strapi's authentication system, which is based on API tokens or on the use of the Users & Permissions plugin.
In some scenarios, it can be useful to have a route publicly available and control the access outside of the normal Strapi authentication system. This can be achieved by setting the auth
configuration parameter of a route to false
:
- Core router with a public route
- Custom router with a public route
- JavaScript
- TypeScript
const { createCoreRouter } = require('@strapi/strapi').factories;
module.exports = createCoreRouter('api::restaurant.restaurant', {
config: {
find: {
auth: false
}
}
});
import { factories } from '@strapi/strapi';
export default factories.createCoreRouter('api::restaurant.restaurant', {
config: {
find: {
auth: false
}
}
});
- JavaScript
- TypeScript
module.exports = {
routes: [
{
method: 'GET',
path: '/articles/customRoute',
handler: 'api::api-name.controllerName.functionName', // or 'plugin::plugin-name.controllerName.functionName' for a plugin-specific controller
config: {
auth: false,
},
},
],
};
export default {
routes: [
{
method: 'GET',
path: '/articles/customRoute',
handler: 'api::api-name.controllerName.functionName', // or 'plugin::plugin-name.controllerName.functionName' for a plugin-specific controller
config: {
auth: false,
},
},
],
};